Once upon a time, JavaScript was a small subsection of web design and web development. Now it represents a large portion of it. Your web browser can even do things that interact out with the web that you would expect to see in a systems language like Java, C or VB.NET.
Some such examples include interfacing with the operating system, interfacing with external devices and getting system information. Strictly speaking, although I refer to these as 'HTML5 APIs' this is actually a bit of a misnomer since they are not actually HTML5 but JavaScript related. They are referred to generally as HTML5 APIs so in this article I too will refer to them by this name.
In this article, I'm going to step into the world of JavaScript and look at some of the new APIs (application programming interfaces) and features that we can use fairly usefully. This article is aimed to be particularly interesting for those who are a bit newer to JavaScript.
This article is designed also just for fun and not necessarily to show you features you may implement in a production product. It takes a lot of it's inspiration and content from the wonderful people at the Mozilla Developer Network (MDN) too, so please be sure to pay them a visit!
An introduction to the navigator
So let's look at the basics of our webpage. We call the code HTML. This is then parsed in to an structure. We call this structure a model and it's a model of objects so it's known as the Document Object Model (DOM). This is an interactive version of the HTML code that can be manipulated much quicker (imagine having to change HTML all the time to get a result). The objects of the DOM are known as elements and these are placed (drawn) on the screen of the computer be it a smartphone, laptop or whatever.
The DOM is redrawn (invalidated) when something changes within it such as text is drawn on an item, but to make the DOM more efficient, and since it follows a tree hierarchy it is already designed to be efficient at manipulation, only the part that has changed is redrawn.
JavaScript refers to the browser itself as what it calls a 'navigator'. The navigator is inside something different to the DOM called the BOM or Browser Object Model. With the BOM, information about the browser, the window etc is available to the JavaScript engine.
This article is looking mainly at the navigator object itself.
Battery API
The battery API is one of the newest ones to come to browsers and it's new to HTML5 browsers. This API gives access to the system battery, so in a laptop or smartphone, the webpage can view information about the battery level, whether the battery is charging and how long it is until it is fully charged. Let's take a look at one example from MDN:
navigator.getBattery().then(function(battery) { alert(battery.charging); });
In the example above, which is inspired by the Mozilla Developer Network sample, the user is told through an alert box whether or not the battery is charging or not (in the form of a true or false).
Let's take a look then.
How can this be used in a JavaScript application you may ask? Well in one way, a website or web app or general application written in JavaScript could reduce the amount of processing that would be done based on the user's battery situation - whether or not it is charging or not. This could also be useful for smartphones where battery levels are something users are very concious of.
Now what about levels? Because it would be useful to know what the level of charge is on the battery. So we can use the same system to obtain the levels:
navigator.getBattery().then(function(battery) { alert(battery.level); });
Clicking the button below will give you the battery level of your computer.
So there you have it, the HTML5 Battery API.
WebSockets API
The next HTML5 API for JavaScript is the WebSockets API. This API opens and closes web sockets, allowing us to enter a two-way communication with a server. This means that live updates are possible using a more lightweight option than Ajax.
Firstly, the premise for the use of the WebSockets API comes from the demand for a more lightweight method of refreshing live content. For a start, it will be integrated into the next version of my BalfBlog content management system.
What this will do for blogging systems like BalfBlog is remove demand from the server and reduce the amount of data being sent backward and forward.
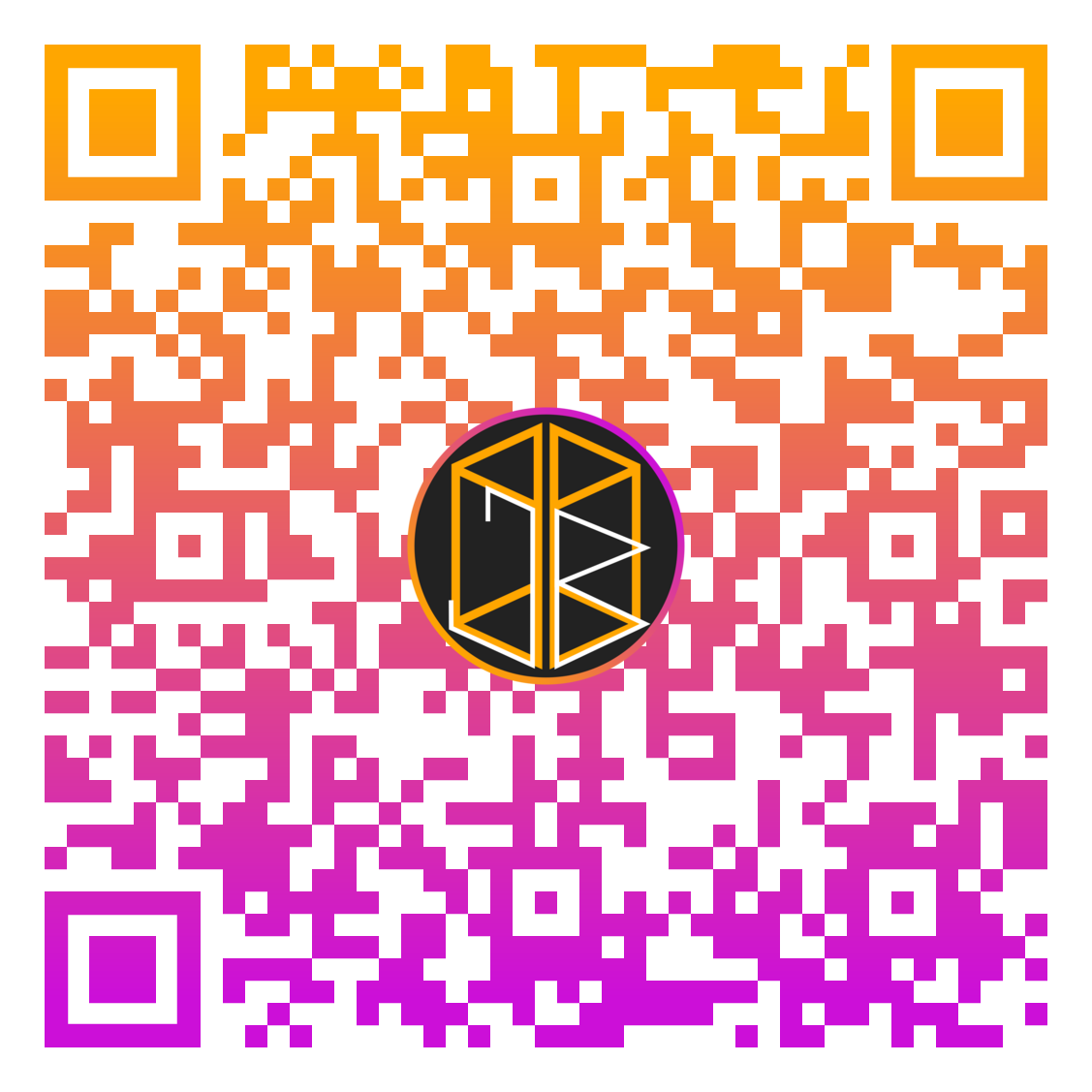